- Download All libraries and file:
I - Windows phone
Necessary
libraries
- GoolgeAds
1. Add GoogleAds dll
- Open folder contain dll file -> right click then check
Unblock if it doesn’t unlock yet.
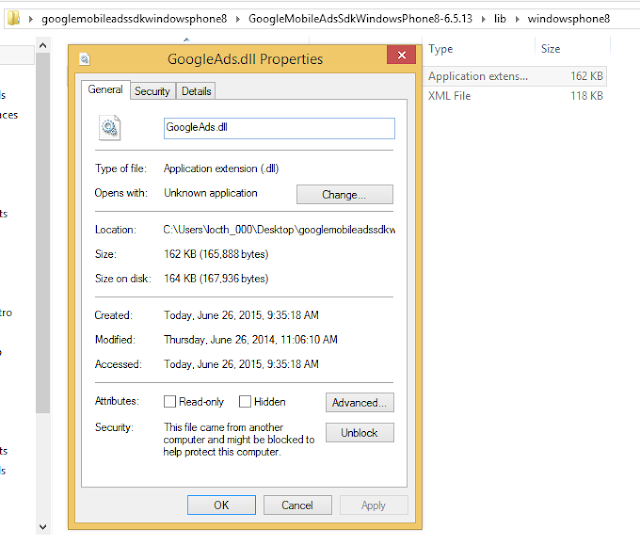
- On your Windows phone project (in Solution
Explorer) chose References tab
->Right click Add References -> Browse…. and target to your GoogleAds dll
file.
2. Create Ad
- There are two type of Admob: AdBanner and Interstitial
Ad
·
Ad Banner:
Create C# Method at MainPage.xaml.cs (in windows phone
project)
public void LoadAD()
{
AdView banner = new AdView
{
AdUnitID = "your app-id",
VerticalAlignment =
System.Windows.VerticalAlignment.Bottom,
HorizontalAlignment =
System.Windows.HorizontalAlignment.Center,
Format = AdFormats.SmartBanner,
Width = App.Current.Host.Content.ActualWidth,
Height = 100
};
AdRequest ad = new AdRequest();
//ad.ForceTesting
= true; if you want to test ad
banner.LoadAd(ad);
banner.Visibility = System.Windows.Visibility.Visible;
DrawingSurfaceBackground.Children.Add(banner);
}
- DrawingSurfaceBackground is a Grid parent in windows phone you can see it at MainPage.xaml
- Then
you can call method LoadAD() to load AdBanner. Example: you can call it in public MainPage() constructor
· Interstitial
Ad
- This ad just call once and
it show full screen.
public void LoadInterstitalAD()
{
AdRequest ad = new AdRequest();
//ad.ForceTesting
= true;
InterstitialAd Ad = new InterstitialAd("your interstitial app-id");
Ad.LoadAd(ad);
Ad.ReceivedAd += (o, e) =>
{
Ad.ShowAd();
};
}
- You
don’t need to add it to parent container like BannerAD. Ad will show it you
call LoadInterstitalAD() method
3. Handle in
C++
- If you want to handle Ad in
your C++ code (cocos2d-x) you must read this post:
II – Android
Necessary
libraries
- google-play-services_lib
- C++ class
1. Add google-play-services_lib
- Open Eclipse and add project, then import google-play-services_lib
- Right click your android project -> chose properties
-> Resource tab -> Android -> at Library click Add button then chose google-play-services_lib
->Apply -> OK
- Then you copy AdmobHelper.cpp and AdmobHelper.h to your
Classes folder and import it to your android project.
2. Create Ad
- Copy code from AppActivity.txt to your AppActivity.java.
It is located in folder: your-android-projc/src/org.cocos2dx.cpp/AppActivity.java
- Enter your app-id to load AD.
3. Handle in C++
- Include AdmobHelper.h
- use: AdmobHelper::ShowAd() to show ad and
AdmobHelper::HideAd() to hide ad
0 Comment:
Post a Comment