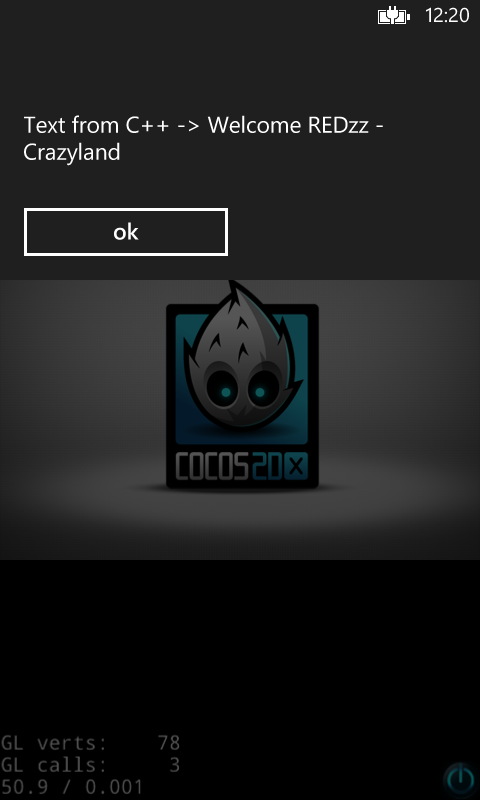
If you code on windows phone with cocos2d-x maybe sometime you need to call method from C# like share link facebook, email, in-app purchase, ads,.... So that I write this tutorial to help someone who want to call C# method from C++. Now let do it!
- First you create
a new project and open wp8 project (I’m using cocos2d-x 3.4).
If you have error like this:
You need do add cocos2d namespace by : using cocos2d; anh then go BUILD -> Clean
Solution
Note: On cocos2d-x 3.3 and lower namespace PhoneDirect3DxamlAppComponent was changed to cocos2d.I’m using cocos 3.4 so I work on name space cocos2d, if you work on lower version name space is PhoneDirect3DxamlAppComponent.
- Open
HelloWorldScene.h we will do something in here:
You know, in cocos2d-x 3.4 C# and C++ use namespace cocos2d together and
we will do in this namespace.
Well, I will create an Interface class and a ref class like
this above class HelloWorld
namespace cocos2d { [Windows::Foundation::Metadata::WebHostHidden] public interface class ICallback { public: virtual void CallFunc(Platform::String ^mess); }; public ref class PlatformCenter sealed { public: PlatformCenter(void); static void setCallback(ICallback ^callback); static void callFunc(Platform::String^ s); }; };
Interface class ICallback will be used on C# and class
PlatformCenter will be used on C++ to call callFunc() from C#.
We need 2 static method:
static void setCallback(ICallback ^callback);
this
use to selCallback in C# like delegate.
static void callFunc(Platform::String^ s);
this use to call callFunc() from C++ with String input.
- Open
HelloWorldScene.cpp and we will code CallFunc method:
Add this code above HelloWorld class
namespace cocos2d { ICallback ^callBack = nullptr; PlatformCenter::PlatformCenter() { } void PlatformCenter::callFunc(Platform::String ^s) { callBack->CallFunc(s); } void PlatformCenter::setCallback(ICallback ^callback) { callBack = callback; } };
- Next we leave to
MainPage.xaml.cs on C#
On name space cocos2d (cocos2d-x 3.3 and lower
namespace PhoneDirect3DxamlAppComponent)
Create new class inherit PhoneApplicationPage class and ICallback interface. If it
doesn’t work, go to BUILD menu and Rebuild Solution
public class CSCallBack : PhoneApplicationPage, ICallback { public CSCallBack() { PlatformCenter.setCallback(this); } public void CallFunc(String s) { this.Dispatcher.BeginInvoke(() => { MessageBox.Show(s); //MessageBox will show string input from C++. }); } }
Then, go to MainPage class
create new instance of CSCallBack in MainPage Constructor
public MainPage() { InitializeComponent(); CSCallBack cs = new CSCallBack(); …... //some codes under }
define new void method in class HelloWorld (in
HelloWorldScene.h)
void CallCSharp();
next we code in this method:
void HelloWorld::CallCSharp() { std::string s ="Text from C++ -> Welcome REDzz - Crazyland"; std::wstring wstr; wstr.assign(s.begin(), s.end()); PlatformCenter::callFunc(ref new Platform::String(wstr.c_str())); }
In this method:
First we will create a string then convert it to wstring and then to Platform::String
Now run and enjoy winning ^^!.
Download Project on Github:
Well, maybe you need to read more infomation about:
[Windows::Foundation::Metadata::WebHostHidden]
ref class and structs
Platform::String
If you have problem when do this tutorial please leave a comment I will try my best to help you ^^. Finally, this post can have some mistake from me (like wrong typing, wrong grammar, ...) please tell me if you see it ^^ Thanks.
Hi there, i have a problem with this line
ReplyDeletePlatformCenter.setCallback(this);
An exception of type 'System.BadImageFormatException' occurred in PhoneDirect3DXamlAppInterop.DLL but was not handled in user code.
Can u help me?
Thanks you for comment :D
DeleteI found some solution for this problem, you can read here:
http://stackoverflow.com/questions/5229310/system-badimageformatexception-could-not-load-file-or-assembly
http://stackoverflow.com/questions/323140/system-badimageformatexception-could-not-load-file-or-assembly-from-installuti
I think it will help you solve problem :), if you have any problem please tell me :)